Phone Authentication - Firebase Database in Android - Custom UI
The easiest way to authenticate users to firebase is by Phone authentication. Here, the one-time code will be sent to user's phone, that will be used by the user for sign-in. We can use FirebaseUI for it to do it in easier way, like in my other post, PhoneAuthentication . But if you would like to do it more custom, this post will help you. Let's begin!!
PreRequisite:
(a) Go to https://console.firebase.google.com/
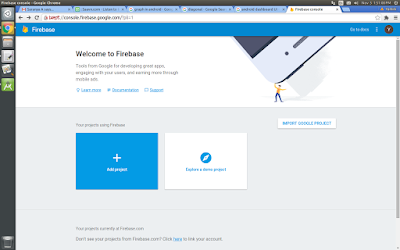
(b) Then click Add Project, a dialog will be prompted. Enter project name and choose country in it and click on Create Project.
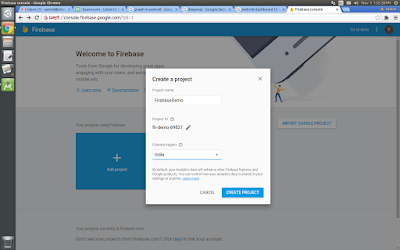
(c) Click on Add Firebase to your Android App, another dialog will be prompted. In that, enter package name and SHA-1 (compulsary for using phone Authentication, Database) and click Register App.
(d) Then download google_services.json file, by clicking download button and put that file in your module's root directory.
(e) Then click on Authentication in side menu and navigate to Sign-in Method. And enable Phone and Save it.
Operational Flow:
Design a layout for user to input his phone number. Also remember that, the phone number should be in the format,
+[countryphonecode][mobileNo.]
Example: +919999988888. Otherwise, it will give an exception. Then sent verification code as SMS to that phone number, that will be verified later using Callbacks. The callback will be invoked in following situations.
(a) Instant Verification - In some cases, the phone number can be instantly verified without sending/receiving SMS.
(b) Auto-Retrieval - On some devices, Google play service will automatically detect and verify the incoming verification SMS, without any user action.
After the verification process is success, we can redirect it to the main page of the app.
Code:
build.gradle (Project level Gradle)
Include the following in dependencies:
classpath 'com.google.gms:google-services:3.1.1'
build.gradle (App-level Gradle)
Include the following in dependencies:
compile 'com.google.firebase:firebase-auth:11.8.0'
And add the following at the bottom:
apply plugin: 'com.google.gms.google-services'
strings.xml:
Add the following string array in strings.xml in values folder. That array is to add country and its phone code.
<string-array name="CountryCodes"> <item>93,AF</item> <item>358 - 18,AX</item> <item>355,AL</item> <item>213,DZ</item> <item>1 - 684,AS</item> <item>376,AD</item> <item>244,AO</item> <item>1 - 264,AI</item> <item>672 ,AQ</item> <item>1 - 268,AG</item> <item>54,AR</item> <item>374,AM</item> <item>297,AW</item> <item>61,AU</item> <item>43,AT</item> <item>994,AZ</item> <item>1 - 242,BS</item> <item>973,BH</item> <item>880,BD</item> <item>1 - 246,BB</item> <item>375,BY</item> <item>32,BE</item> <item>501,BZ</item> <item>229,BJ</item> <item>1 - 441,BM</item> <item>975,BT</item> <item>591,BO</item> <item>387,BA</item> <item>267,BW</item> <item>91,BV</item> <item>55,BR</item> <item>246,IO</item> <item>673,BN</item> <item>359,BG</item> <item>226,BF</item> <item>257,BI</item> <item>855,KH</item> <item>237,CM</item> <item>1,CA</item> <item>238,CV</item> <item>1 - 345,KY</item> <item>236,CF</item> <item>235,TD</item> <item>56,CL</item> <item>86,CN</item> <item>61,CX</item> <item>61,CC</item> <item>57,CO</item> <item>269,KM</item> <item>242,CG</item> <item>243,CD</item> <item>682,CK</item> <item>506,CR</item> <item>225,CI</item> <item>385,HR</item> <item>53,CU</item> <item>357,CY</item> <item>420,CZ</item> <item>45,DK</item> <item>253,DJ</item> <item>1 - 767,DM</item> <item>1 - 809, - 1 ,DO</item> <item>593,EC</item> <item>20,EG</item> <item>503,SV</item> <item>240,GQ</item> <item>291,ER</item> <item>372,EE</item> <item>251,ET</item> <item>500,FK</item> <item>298,FO</item> <item>679,FJ</item> <item>358,FI</item> <item>33,FR</item> <item>594,GF</item> <item>689,PF</item> <item>91,TF</item> <item>241,GA</item> <item>220,GM</item> <item>995,GE</item> <item>49,DE</item> <item>233,GH</item> <item>350,GI</item> <item>30,GR</item> <item>299,GL</item> <item>1 - 473,GD</item> <item>590,GP</item> <item>1 - 671,GU</item> <item>502,GT</item> <item>44,GG</item> <item>224,GN</item> <item>245,GW</item> <item>592,GY</item> <item>509,HT</item> <item>91,HM</item> <item>379,VA</item> <item>504,HN</item> <item>852,HK</item> <item>36,HU</item> <item>91,IN</item> <item>354,IS</item> <item>62,ID</item> <item>98,IR</item> <item>964,IQ</item> <item>353,IE</item> <item>44,IM</item> <item>972,IL</item> <item>39,IT</item> <item>1 - 876,JM</item> <item>81,JP</item> <item>44,JE</item> <item>962,JO</item> <item>7,KZ</item> <item>254,KE</item> <item>686,KI</item> <item>850,KP</item> <item>82,KR</item> <item>965,KW</item> <item>996,KG</item> <item>856,LA</item> <item>371,LV</item> <item>961,LB</item> <item>266,LS</item> <item>231,LR</item> <item>218,LY</item> <item>423,LI</item> <item>370,LT</item> <item>352,LU</item> <item>853,MO</item> <item>389,MK</item> <item>261,MG</item> <item>265,MW</item> <item>60,MY</item> <item>960,MV</item> <item>223,ML</item> <item>356,MT</item> <item>692,MH</item> <item>596,MQ</item> <item>222,MR</item> <item>230,MU</item> <item>262,YT</item> <item>52,MX</item> <item>691,FM</item> <item>373,MD</item> <item>377,MC</item> <item>976,MN</item> <item>382,ME</item> <item>1 – 664,MS</item> <item>212,MA</item> <item>258,MZ</item> <item>95,MM</item> <item>264,NA</item> <item>674,NR</item> <item>977,NP</item> <item>31,NL</item> <item>599,AN</item> <item>687,NC</item> <item>64,NZ</item> <item>505,NI</item> <item>227,NE</item> <item>234,NG</item> <item>683,NU</item> <item>672,NF</item> <item>670,MP</item> <item>47,NO</item> <item>968,OM</item> <item>92,PK</item> <item>680,PW</item> <item>970,PS</item> <item>507,PA</item> <item>675,PG</item> <item>595,PY</item> <item>51,PE</item> <item>63,PH</item> <item>64,PN</item> <item>48,PL</item> <item>351,PT</item> <item>1 787,PR</item> <item>974,QA</item> <item>262,RE</item> <item>40,RO</item> <item>7,RU</item> <item>250,RW</item> <item>590,BL</item> <item>290,SH</item> <item>1 – 869,KN</item> <item>758,LC</item> <item>590,MF</item> <item>508,PM</item> <item>1 - 784,VC</item> <item>685,WS</item> <item>378,SM</item> <item>239,ST</item> <item>966,SA</item> <item>221,SN</item> <item>381,RS</item> <item>248,SC</item> <item>232,SL</item> <item>65,SG</item> <item>421,SK</item> <item>386,SI</item> <item>677,SB</item> <item>252,SO</item> <item>27,ZA</item> <item>500,GS</item> <item>34,ES</item> <item>94,LK</item> <item>249,SD</item> <item>597,SR</item> <item>47,SJ</item> <item>268,SZ</item> <item>46,SE</item> <item>41,CH</item> <item>963,SY</item> <item>886,TW</item> <item>992,TJ</item> <item>255,TZ</item> <item>66,TH</item> <item>670,TL</item> <item>228,TG</item> <item>690,TK</item> <item>676,TO</item> <item>1 - 868,TT</item> <item>216,TN</item> <item>90,TR</item> <item>993,TM</item> <item>1 - 649,TC</item> <item>688,TV</item> <item>256,UG</item> <item>380,UA</item> <item>971,AE</item> <item>44,GB</item> <item>1,US</item> <item>91,UM</item> <item>598,UY</item> <item>998,UZ</item> <item>678,VU</item> <item>58,VE</item> <item>84,VN</item> <item>1 284,VG</item> <item>1 340,VI</item> <item>681,WF</item> <item>212,EH</item> <item>967,YE</item> <item>260,ZM</item> <item>260,ZW</item> </string-array>
registration.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/white" android:orientation="vertical"> <LinearLayout android:id="@+id/registerLayout" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_margin="16dp" android:gravity="top|center" android:orientation="vertical" android:visibility="visible"> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="15dp" android:gravity="center" android:orientation="vertical" android:paddingBottom="15dp"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center_horizontal" android:text="Welcome to Firebase" android:textColor="@color/colorPrimaryDark" android:textSize="22sp" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_marginTop="15dp" android:gravity="center_horizontal" android:text="SMS will be sent to your mobile number" android:textSize="12sp" /> <Spinner android:id="@+id/countryList" android:layout_width="match_parent" android:layout_height="40dp" android:layout_marginLeft="6dp" android:layout_marginRight="6dp" android:layout_marginTop="5dp" /> <View android:layout_width="fill_parent" android:layout_height="1dp" android:layout_marginLeft="6dp" android:layout_marginRight="6dp" android:background="#e6e6e6" /> <LinearLayout android:id="@+id/phone_layout" android:layout_width="fill_parent" android:layout_height="40dp" android:layout_marginLeft="6dp" android:layout_marginRight="6dp" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:id="@+id/countryCode" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="0.3" android:background="@android:color/transparent" android:gravity="left|center_vertical" android:text="+" android:textColor="#505050" android:textSize="14sp" /> <EditText android:id="@+id/phonenumber" android:layout_width="0dp" android:layout_height="39dp" android:layout_marginLeft="10dp" android:layout_weight="1.7" android:background="@android:color/transparent" android:hint="Phone Number" android:inputType="phone" android:maxLength="13" android:maxLines="1" android:singleLine="true" android:textSize="14sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <View android:layout_width="0dp" android:layout_height="1dp" android:layout_weight="0.3" android:background="#e6e6e6" android:visibility="visible" /> <View android:layout_width="0dp" android:layout_height="1dp" android:layout_marginLeft="10dp" android:layout_weight="1.7" android:background="#e6e6e6" android:visibility="visible" /> </LinearLayout> </LinearLayout> <Button android:id="@+id/next" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginLeft="6dp" android:layout_marginRight="6dp" android:layout_marginTop="25dp" android:background="@color/colorPrimaryDark" android:text="Next" android:textColor="#fff" android:textSize="18sp" tools:ignore="UnusedAttribute" /> </LinearLayout> </LinearLayout> <include layout="@layout/verification" android:visibility="gone" /> <RelativeLayout android:id="@+id/signedLayout" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_above="@+id/signOut" android:layout_marginBottom="10dp" android:gravity="center" android:text="You've signed in successfully!"/> <Button android:id="@+id/signOut" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" android:layout_marginLeft="6dp" android:layout_marginRight="6dp" android:layout_marginTop="25dp" android:background="@color/colorPrimaryDark" android:text="Sign Out" android:textColor="#fff" android:textSize="18sp" tools:ignore="UnusedAttribute" /> </RelativeLayout> </LinearLayout>
verification.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/verifyLayout" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="vertical"> <TextView android:id="@+id/activation_verifyno" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:gravity="center_horizontal" android:text="Verify" android:textColor="@color/colorPrimaryDark" android:textSize="20sp" /> <TextView android:id="@+id/senttsms" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="12dp" android:text="Waiting for SMS" android:textAppearance="@android:style/TextAppearance.Small" /> <EditText android:id="@+id/inputCode" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_marginTop="20dp" android:ems="10" android:gravity="center" android:hint="- - - - - -" android:inputType="number" android:maxLength="6" android:maxLines="1" android:paddingLeft="5dp" android:textColorHint="@color/colorPrimary"> </EditText> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:text="Enter 6 digit Code" android:textAppearance="@android:style/TextAppearance.Small"/> <Button android:id="@+id/codeVerify" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="20dp" android:background="@color/colorPrimaryDark" android:text="Submit" android:textAppearance="@android:style/TextAppearance.Medium" android:textColor="@color/white" android:visibility="visible" /> <Button android:id="@+id/resendsms" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="left" android:layout_marginLeft="20dp" android:layout_marginTop="20dp" android:background="@android:color/transparent" android:drawablePadding="10dp" android:gravity="left|center_vertical" android:padding="5dp" android:drawableLeft="@drawable/mail" android:text="Resend SMS" android:textAppearance="@android:style/TextAppearance.Small"/> </LinearLayout>
MainActivity.java
public class MainActivity extends AppCompatActivity implements View.OnClickListener { EditText phone, codeInput; Button next, codeVerify, resend, signOut; LinearLayout registerLayout, verifyLayout; RelativeLayout signedLayout; Spinner cityList; TextView countryCode; private PhoneAuthProvider.OnVerificationStateChangedCallbacks mCallbacks; private static final String TAG = "PhoneAuthentication"; private FirebaseAuth mAuth; private boolean mVerificationInProgress = false; private static final int STATE_INITIALIZED = 1; private static final int STATE_CODE_SENT = 2; private static final int STATE_VERIFY_FAILED = 3; private static final int STATE_VERIFY_SUCCESS = 4; private static final int STATE_SIGNIN_FAILED = 5; private static final int STATE_SIGNIN_SUCCESS = 6; private String mVerificationId; private PhoneAuthProvider.ForceResendingToken mResendToken; private ArrayList<String> country_code = new ArrayList<>(); private ArrayList<String> country = new ArrayList<>(); private ArrayList<String> country_withCode = new ArrayList<>(); private ArrayList<String> country_ = new ArrayList<>(); private String countryname, code; private static final String KEY_VERIFY_IN_PROGRESS = "key_verify_in_progress"; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.registration); phone = findViewById(R.id.phonenumber); next = findViewById(R.id.next); codeVerify = findViewById(R.id.codeVerify); registerLayout = findViewById(R.id.registerLayout); verifyLayout = findViewById(R.id.verifyLayout); cityList = findViewById(R.id.countryList); countryCode = findViewById(R.id.countryCode); codeInput = findViewById(R.id.inputCode); resend = findViewById(R.id.resendsms); signedLayout = findViewById(R.id.signedLayout); signOut = findViewById(R.id.signOut); mAuth = FirebaseAuth.getInstance(); getCountry(); CallCountryListService(); loadSpinner(); mCallbacks = new PhoneAuthProvider.OnVerificationStateChangedCallbacks() { @Override public void onVerificationCompleted(PhoneAuthCredential credential) { // This callback will be invoked in two situations: // 1 - Instant verification. In some cases the phone number can be instantly // verified without needing to send or enter a verification code. // 2 - Auto-retrieval. On some devices Google Play services can automatically // detect the incoming verification SMS and perform verification without // user action. Log.d(TAG, "onVerificationCompleted:" + credential); mVerificationInProgress = false; updateUI(STATE_VERIFY_SUCCESS, credential); signInWithPhoneAuthCredential(credential); } @Override public void onVerificationFailed(FirebaseException e) { // This callback is invoked in an invalid request for verification is made, // for instance if the the phone number format is not valid. Log.w(TAG, "onVerificationFailed", e); mVerificationInProgress = false; e.printStackTrace(); if (e instanceof FirebaseAuthInvalidCredentialsException) { phone.setError("Invalid phone number!!"); } else if (e instanceof FirebaseTooManyRequestsException) { Snackbar.make(findViewById(android.R.id.content), "Quota exceeded.", Snackbar.LENGTH_SHORT).show(); } updateUI(STATE_VERIFY_FAILED); } @Override public void onCodeSent(String verificationId, PhoneAuthProvider.ForceResendingToken token) { // The SMS verification code has been sent to the provided phone number, we // now need to ask the user to enter the code and then construct a credential // by combining the code with a verification ID. Log.d(TAG, "onCodeSent:" + verificationId); // Save verification ID and resending token so we can use them later mVerificationId = verificationId; mResendToken = token; updateUI(STATE_CODE_SENT); } }; next.setOnClickListener(this); codeVerify.setOnClickListener(this); resend.setOnClickListener(this); signOut.setOnClickListener(this); } private String getCountry() { try { TelephonyManager manager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE); // getNetworkCountryIso String CountryID = manager.getSimCountryIso().toUpperCase(); Locale loc = new Locale("", CountryID); countryname = loc.getDisplayCountry(); } catch (Exception e) { e.printStackTrace(); } return countryname; } private void CallCountryListService() { String[] rl = this.getResources().getStringArray(R.array.CountryCodes); String CountryID; for (String aRl : rl) { String[] g = aRl.split(","); CountryID = g[1].trim().toUpperCase(); if (g[1].trim().equals(CountryID.trim())) { Locale loc = new Locale("", CountryID); country.add(loc.getDisplayCountry()); country_code.add(g[0]); } } } private void loadSpinner() { int SelectPos = 0; int size = country.size(); Iterator<String> iterator = country.iterator(); Iterator<String> iterator1 = country_code.iterator(); while (iterator.hasNext() && iterator1.hasNext()) { String FirstName = iterator.next(); String LastName = iterator1.next(); country_withCode.add(FirstName + " " + "(+" + LastName + ")"); country_.add(FirstName); } ArrayAdapter<String> spinnerArrayAdapter = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, country_); spinnerArrayAdapter .setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); cityList.setAdapter(spinnerArrayAdapter); for (int i = 0; i < size; i++) { if (country.get(i).equals(countryname)) { SelectPos = i; break; } } cityList.setSelection(SelectPos); cityList.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2, long arg3) { code = country_code.get(arg2); countryCode.setText("+" + code); System.out.println("selectedcountry--->" + arg2); } @Override public void onNothingSelected(AdapterView<?> arg0) { } }); } private void updateUI(int uiState) { updateUI(uiState, mAuth.getCurrentUser(), null); } private void updateUI(FirebaseUser user) { if (user != null) { updateUI(STATE_SIGNIN_SUCCESS, user); } else { updateUI(STATE_INITIALIZED); } } private void updateUI(int uiState, FirebaseUser user) { updateUI(uiState, user, null); } private void signInWithPhoneAuthCredential(PhoneAuthCredential credential) { mAuth.signInWithCredential(credential) .addOnCompleteListener(this, new OnCompleteListener<AuthResult>() { @Override public void onComplete(@NonNull Task<AuthResult> task) { if (task.isSuccessful()) { // Sign in success, update UI with the signed-in user's information Log.d(TAG, "signInWithCredential:success"); FirebaseUser user = task.getResult().getUser(); updateUI(STATE_SIGNIN_SUCCESS, user); } else { // Sign in failed, display a message and update the UI Log.w(TAG, "signInWithCredential:failure", task.getException()); if (task.getException() instanceof FirebaseAuthInvalidCredentialsException) { codeInput.setError("Invalid code."); } updateUI(STATE_SIGNIN_FAILED); } } }); } private void updateUI(int uiState, PhoneAuthCredential cred) { updateUI(uiState, null, cred); } private void updateUI(int uiState, FirebaseUser user, PhoneAuthCredential cred) { switch (uiState) { case STATE_INITIALIZED: // Initialized state, show only the phone number field and start button registerLayout.setVisibility(View.VISIBLE); verifyLayout.setVisibility(View.GONE); signedLayout.setVisibility(View.GONE); break; case STATE_CODE_SENT: // Code sent state, show the verification field, the registerLayout.setVisibility(View.GONE); verifyLayout.setVisibility(View.VISIBLE); signedLayout.setVisibility(View.GONE); break; case STATE_VERIFY_FAILED: // Verification has failed, show all options break; case STATE_VERIFY_SUCCESS:
// Verification has succeeded, proceed to firebase sign in if (cred != null) { if (cred.getSmsCode() != null) { codeInput.setText(cred.getSmsCode()); } } registerLayout.setVisibility(View.GONE); verifyLayout.setVisibility(View.GONE); signedLayout.setVisibility(View.VISIBLE); break; case STATE_SIGNIN_FAILED: // No-op, handled by sign-in check break; case STATE_SIGNIN_SUCCESS: // Np-op, handled by sign-in check registerLayout.setVisibility(View.GONE); verifyLayout.setVisibility(View.GONE); signedLayout.setVisibility(View.VISIBLE); break; } } private boolean validatePhoneNumber() { String phoneNumber = phone.getText().toString(); String phoneCode = countryCode.getText().toString(); if (TextUtils.isEmpty(phoneNumber)) { phone.setError("Invalid phone number."); return false; } if (TextUtils.isEmpty(phoneCode)) { countryCode.setError("Invalid Country Code."); return false; } return true; } private void startPhoneNumberVerification(String phoneNumber) { PhoneAuthProvider.getInstance().verifyPhoneNumber( phoneNumber, 60, TimeUnit.SECONDS, this, mCallbacks); mVerificationInProgress = true; } private void verifyPhoneNumberWithCode(String verificationId, String code) { PhoneAuthCredential credential = PhoneAuthProvider.getCredential(verificationId, code); signInWithPhoneAuthCredential(credential); } private void resendVerificationCode(String phoneNumber, PhoneAuthProvider.ForceResendingToken token) { PhoneAuthProvider.getInstance().verifyPhoneNumber( phoneNumber, 60, TimeUnit.SECONDS, this, mCallbacks, token); } private void signOut() { mAuth.signOut(); updateUI(STATE_INITIALIZED); } @Override public void onStart() { super.onStart(); // Check if user is signed in (non-null) and update UI accordingly. FirebaseUser currentUser = mAuth.getCurrentUser(); updateUI(currentUser); if (mVerificationInProgress && validatePhoneNumber()) { startPhoneNumberVerification("+"+code+phone.getText().toString()); } } @Override protected void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); outState.putBoolean(KEY_VERIFY_IN_PROGRESS, mVerificationInProgress); } @Override protected void onRestoreInstanceState(Bundle savedInstanceState) { super.onRestoreInstanceState(savedInstanceState); mVerificationInProgress = savedInstanceState.getBoolean(KEY_VERIFY_IN_PROGRESS); } /* To hide keyboard regarding to view */ public void hideKeyboard(View view) { try { InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE); inputMethodManager.hideSoftInputFromWindow(view.getWindowToken(), 0); } catch (Exception e) { e.printStackTrace(); } } @Override public void onClick(View v) { switch (v.getId()){ case R.id.next: hideKeyboard(next); if (!validatePhoneNumber()) { return; } startPhoneNumberVerification("+"+code+phone.getText().toString()); break; case R.id.codeVerify: hideKeyboard(codeVerify); String tempcode = codeInput.getText().toString(); if (TextUtils.isEmpty(tempcode)) { codeInput.setError("Cannot be empty."); return; } verifyPhoneNumberWithCode(mVerificationId, tempcode); break; case R.id.resendsms: hideKeyboard(resend); resendVerificationCode("+"+code+phone.getText().toString(), mResendToken); break; case R.id.signOut: hideKeyboard(signOut); signOut(); break; } } }
Run Application:
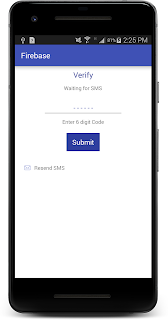
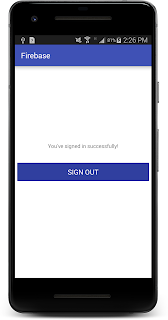
Note:
Also remember that the Phone Authentication will not work in Emulator, it requires Physical device as per Document.
Comments
Post a Comment